Avoid Coding Headaches: Mastering The Off-by-One Error
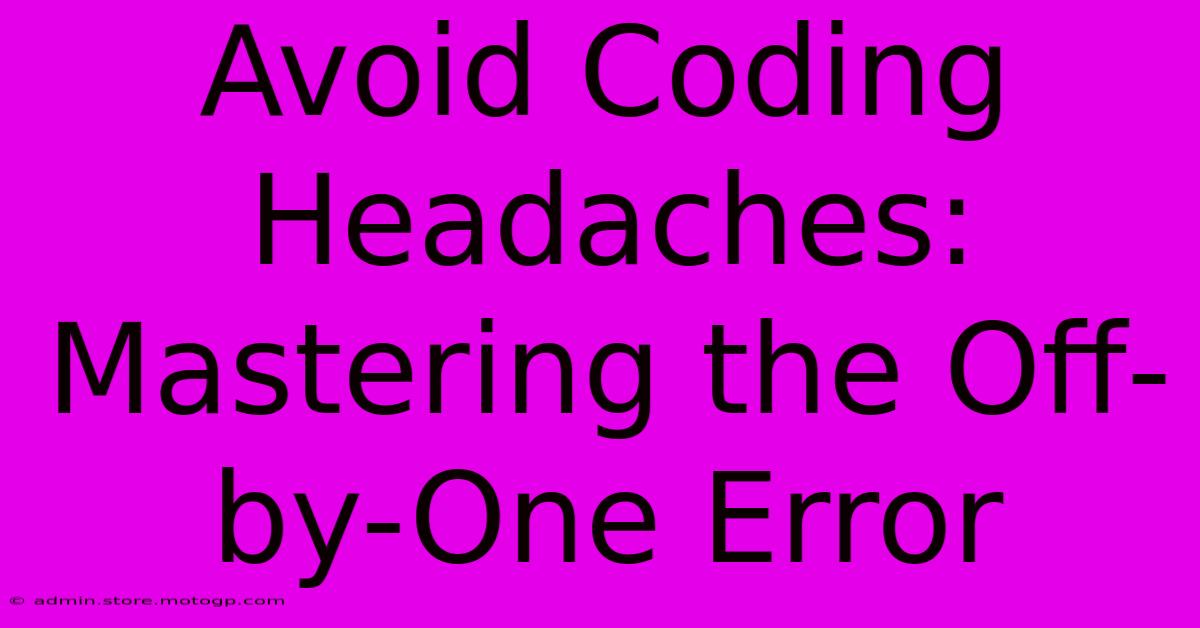
Table of Contents
Avoid Coding Headaches: Mastering the Off-by-One Error
The off-by-one error, or OBOE, is a common and frustrating bug in programming. It's the kind of error that can send even seasoned developers into a debugging frenzy, wasting precious time and energy. Understanding the root causes of OBOEs and implementing preventative measures is crucial for writing cleaner, more efficient, and less error-prone code. This article will guide you through the intricacies of this pervasive problem, equipping you with the knowledge to avoid it altogether.
What is an Off-by-One Error?
An off-by-one error occurs when a loop iterates one time too many or one time too few. This seemingly small discrepancy can lead to significant problems, ranging from incorrect calculations to program crashes. The error stems from a mismatch between the intended range of iteration and the actual number of iterations performed.
Example: Imagine you want to print numbers 1 through 5. An off-by-one error might result in printing numbers 1 through 4 or 1 through 6.
Common Causes of Off-by-One Errors
Several programming practices increase the likelihood of OBOEs:
1. Incorrect Loop Conditions:
This is the most frequent culprit. Failing to correctly define the loop's starting and ending conditions is a recipe for disaster. For example, using <
instead of <=
(or vice versa) when iterating through an array can easily lead to an OBOE.
Example (Incorrect):
for (int i = 0; i < array.length; i++) { // Misses the last element
// ... code ...
}
Example (Correct):
for (int i = 0; i < array.length; i++) { //Iterates through all elements
// ... code ...
}
2. Confusion with 0-based Indexing:
Many programming languages use 0-based indexing for arrays and lists. Forgetting this can cause an OBOE. The first element is at index 0, not 1. Confusing this often results in accessing an element outside the array's bounds, leading to runtime errors.
3. Incorrect Boundary Handling:
Problems arise when dealing with edge cases – the beginning and end of a data structure or range. Carefully considering these boundaries is vital to prevent OBOEs. Pay close attention to whether you need inclusive or exclusive boundaries.
4. Off-by-One Errors in Recursive Functions:
Recursive functions can also fall prey to OBOEs. Incorrect base cases or recursive calls that don't properly decrement or increment the counter can lead to infinite loops or incorrect results.
Best Practices to Avoid Off-by-One Errors
Preventing OBOEs requires careful planning and attention to detail:
- Double-check loop conditions: Before running a loop, meticulously verify the start and end conditions, paying close attention to whether you need
<
,<=
,>
, or>=
. - Understand 0-based indexing: Always remember that arrays and lists typically begin at index 0.
- Use debugging tools: Debuggers allow you to step through your code line by line, inspecting variables and identifying the source of the error.
- Test thoroughly: Comprehensive testing, including edge cases, is critical in uncovering OBOEs.
- Code Reviews: Having another developer review your code can help catch subtle errors like OBOEs that you might have overlooked.
- Clear Comments: Adding comments to your code, especially around loops and boundary conditions, can make it easier to understand and debug later.
- Use descriptive variable names: Well-named variables clarify their purpose and reduce ambiguity, minimizing the risk of off-by-one errors related to index management.
Conclusion
Off-by-one errors are a common but avoidable source of bugs. By understanding their causes and implementing the best practices outlined above, you can significantly improve the quality and reliability of your code, saving yourself countless hours of debugging frustration. Remember, careful planning, thorough testing, and attention to detail are key to mastering this pervasive programming challenge.
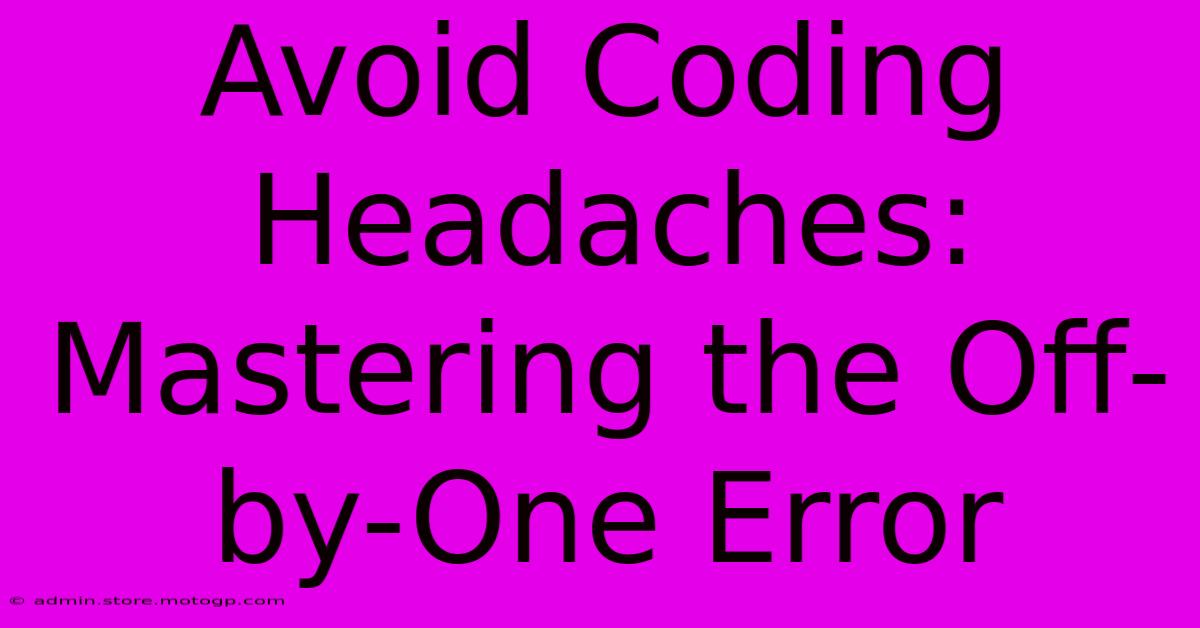
Thank you for visiting our website wich cover about Avoid Coding Headaches: Mastering The Off-by-One Error. We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and dont miss to bookmark.
Featured Posts
-
The X Factor Unlocking The Explosive Potential Of Stock Warrants With Our Exclusive List
Feb 09, 2025
-
The Great Jewelry Orthography Debate Jewellers Vs Jewelers A Linguistic Odyssey
Feb 09, 2025
-
Is Cloak And Dagger 1984 The Ultimate 80s Kids Adventure
Feb 09, 2025
-
Olympic Valley Ca Your Us Basecamp For Adventure
Feb 09, 2025
-
58763 Where Community Thrives In New Town Nd
Feb 09, 2025