Simplify VBA Form Development: Using Variables For Labels And Textboxes
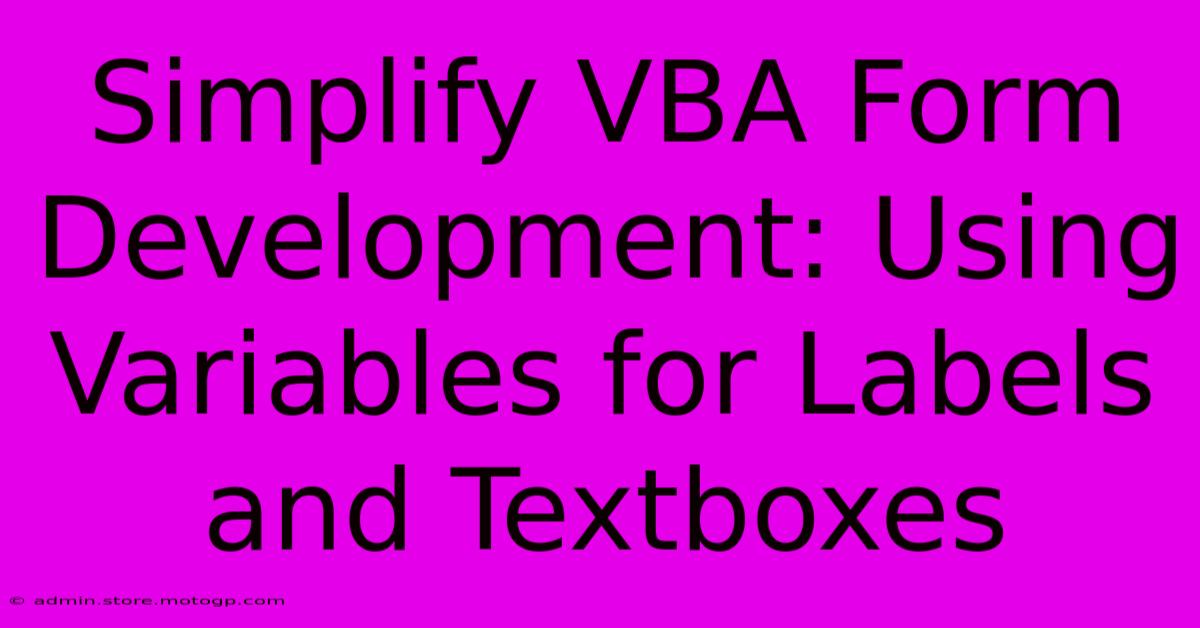
Table of Contents
Simplify VBA Form Development: Using Variables for Labels and Textboxes
Developing user forms in VBA can sometimes feel like navigating a maze. Keeping track of individual controls, especially when dealing with numerous text boxes and labels, can quickly become overwhelming and error-prone. This article demonstrates a streamlined approach to VBA form development by using variables to manage your labels and textboxes, significantly improving code readability, maintainability, and reducing the chance of errors.
The Problem with Direct Control References
Let's consider a simple scenario: you're building a form to collect customer information. Without using variables, your VBA code might look something like this:
Private Sub CommandButton1_Click()
'Directly referencing controls
MsgBox "Customer Name: " & Me.TextBox1.Value & vbCrLf & _
"Customer Address: " & Me.TextBox2.Value & vbCrLf & _
"Customer Phone: " & Me.TextBox3.Value
End Sub
This approach works, but it's fragile. If you rearrange controls, add or remove them, you'll need to meticulously update your code. Imagine a more complex form with dozens of controls—the maintenance nightmare becomes apparent!
The Power of Variables: A More Elegant Solution
By using variables to represent your controls, you dramatically increase the robustness and clarity of your code. Here's how you can refactor the above example:
Private Sub CommandButton1_Click()
Dim txtCustomerName As MSForms.TextBox
Dim txtCustomerAddress As MSForms.TextBox
Dim txtCustomerPhone As MSForms.TextBox
Set txtCustomerName = Me.TextBox1
Set txtCustomerAddress = Me.TextBox2
Set txtCustomerPhone = Me.TextBox3
MsgBox "Customer Name: " & txtCustomerName.Value & vbCrLf & _
"Customer Address: " & txtCustomerAddress.Value & vbCrLf & _
"Customer Phone: " & txtCustomerPhone.Value
'Clean up object variables
Set txtCustomerName = Nothing
Set txtCustomerAddress = Nothing
Set txtCustomerPhone = Nothing
End Sub
This improved code is far easier to read and understand. The variable names clearly indicate the purpose of each control. More importantly, if you later decide to rename or rearrange your text boxes, you only need to update the Set
statements. The rest of your code remains untouched.
Extending the Approach to Labels
The same principle applies to labels. Instead of referencing labels directly (e.g., Me.Label1.Caption
), assign them to variables:
Private Sub UserForm_Initialize()
Dim lblCustomerName As MSForms.Label
Set lblCustomerName = Me.Label1
lblCustomerName.Caption = "Customer Name:"
' ...similarly for other labels...
End Sub
This makes it easy to dynamically update label captions or control their visibility based on your application's logic.
Benefits of Using Variables for VBA Form Controls
- Improved Readability: Code becomes significantly easier to understand and maintain.
- Reduced Errors: Minimizes the risk of typos and inconsistencies when referencing controls.
- Enhanced Flexibility: Facilitates easier modification and expansion of your forms.
- Better Maintainability: Changes to the form's layout have a minimal impact on the code.
- Increased Reusability: You can encapsulate code related to a specific group of controls within a subroutine or function.
Best Practices
- Descriptive Variable Names: Choose names that accurately reflect the control's purpose.
- Error Handling: Include error handling to gracefully manage situations where a control might not be found.
- Object Variable Cleanup: Always use
Set objectVariable = Nothing
to release the object from memory, particularly when dealing with many controls.
By adopting this simple technique of using variables for your labels and textboxes, you’ll dramatically improve the efficiency and maintainability of your VBA form development. Spend less time debugging and more time building powerful and robust applications.
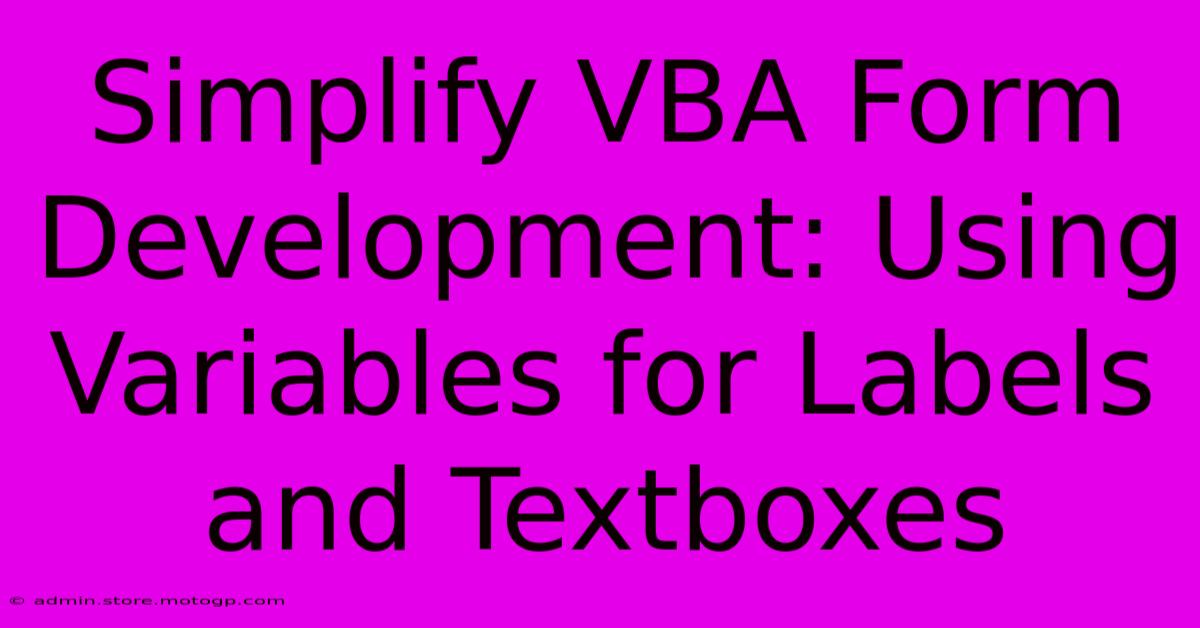
Thank you for visiting our website wich cover about Simplify VBA Form Development: Using Variables For Labels And Textboxes. We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and dont miss to bookmark.
Featured Posts
-
Worshiped Or Worshipped The Ultimate Guide To Proper Usage And Spiritually Enriching Worship
Feb 06, 2025
-
Unlock The Secret Uncover The Ideal Bookmark Size For Your Booming Business
Feb 06, 2025
-
Blooms In Harmony Explore The Art Of Selecting Flowers For Perfect Wedding Centerpieces
Feb 06, 2025
-
Fall Into Fashion Bold And Beautiful Colors To Elevate Your Style
Feb 06, 2025
-
A Canvas Of Color Explore The Artistic Shades Of Baby Breath
Feb 06, 2025