The Ultimate Guide To Squashing Off-by-One Bugs
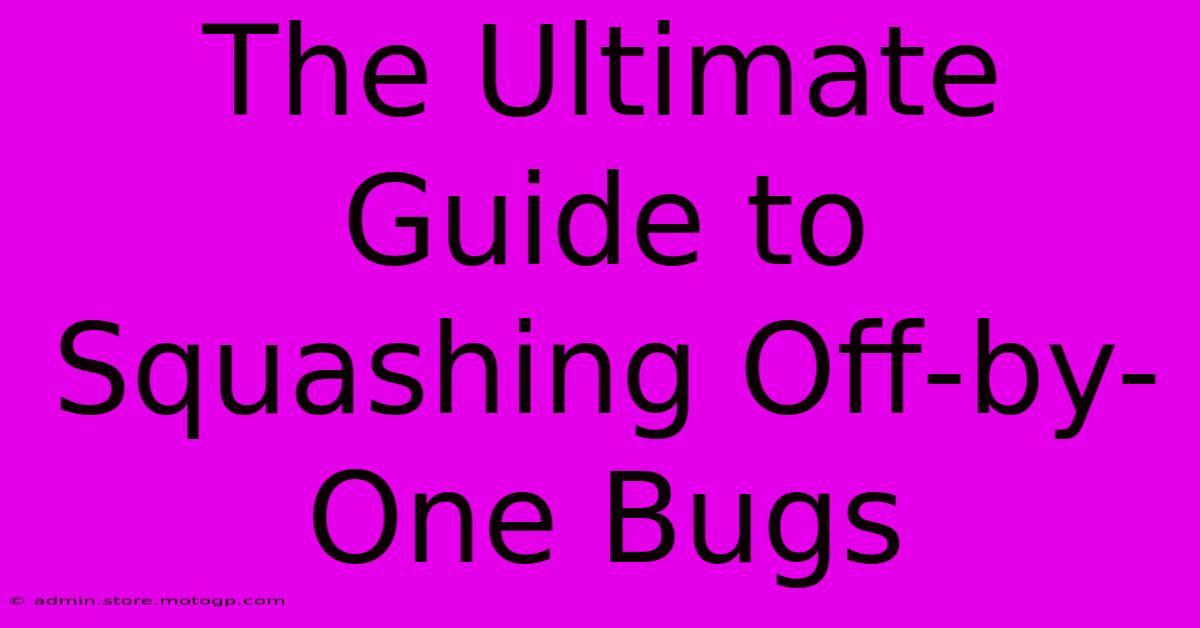
Table of Contents
The Ultimate Guide to Squashing Off-by-One Bugs
Off-by-one errors, those insidious little bugs that plague programmers, are a common source of frustration. They're subtle, often hidden deep within loops and array accesses, and can manifest in unexpected ways. This guide will arm you with the knowledge and techniques to effectively identify, understand, and eliminate these pesky errors once and for all.
Understanding the Off-by-One Error
An off-by-one error, often abbreviated as OBOE, occurs when a loop iterates one time too many or one time too few, or when an array index is accessed outside its valid bounds. These seemingly minor discrepancies can lead to incorrect calculations, unexpected program behavior, or even crashes.
Common Scenarios:
- Looping too many times: This often happens when the loop termination condition is incorrect, leading to an extra iteration.
- Looping too few times: This usually stems from an incorrect starting condition or an early exit from the loop.
- Array index out of bounds: Accessing an element beyond the array's allocated size (either at the beginning or end) can lead to crashes or unpredictable results. This is particularly common in languages without built-in bounds checking.
Identifying Off-by-One Errors: The Detective's Toolkit
Debugging OBOEs requires a methodical approach. Here are some effective strategies:
1. Careful Code Review:
- Trace execution: Manually step through your code, paying close attention to loop counters and array indices. Use a debugger to visually inspect variable values at each step.
- Check boundary conditions: Examine the initial and final states of your loops and array accesses. Pay special attention to the conditions that determine loop termination or array access limits.
- Simplify and test: Break down complex loops into smaller, more manageable units. Test each unit independently to isolate the source of the error.
2. Assertions and Logging:
- Strategic assertions: Insert assertions into your code to verify loop counters and array indices at critical points. Assertions will help you catch errors early in the development process, before they cause larger problems. For example,
assert(i >= 0 && i < array.length);
- Meaningful logging: Log important variables and loop counters to track their values during execution. This detailed logging can help pinpoint the exact location where the off-by-one error occurs.
3. Static Analysis Tools:
Modern IDEs and static analysis tools can automatically detect potential off-by-one errors. These tools often flag suspicious code patterns that might indicate an OBOE. Leveraging these tools can significantly reduce debugging time.
Preventing Off-by-One Errors: Proactive Strategies
Preventing OBOEs is far more efficient than debugging them. Here are some preventative measures:
1. Consistent Indexing:
- Zero-based indexing: Always remember that many programming languages use zero-based indexing for arrays. The first element is at index 0, the second at index 1, and so on.
- Clear conventions: Establish clear conventions for loop counters and array indices. Use meaningful variable names and consistent style to minimize ambiguity.
2. Robust Loop Constructs:
- Iterators: Use iterators whenever possible, as they often handle indexing details automatically, reducing the risk of OBOEs.
- Range-based loops: Languages like C++ and Python offer range-based loops, which simplify iteration and reduce the likelihood of index errors. These loops automatically manage the loop counter, eliminating a common source of off-by-one errors.
3. Thorough Testing:
- Boundary condition testing: Always test your code with boundary conditions. Pay special attention to the extreme values of loop counters and array indices. This includes testing empty arrays, single-element arrays, and arrays with many elements.
- Comprehensive test cases: Develop a comprehensive suite of test cases to cover a wide range of inputs and scenarios.
Conclusion: Mastering the Art of Off-by-One Avoidance
Off-by-one errors are common but avoidable. By combining careful coding practices, diligent debugging techniques, and robust testing strategies, you can significantly reduce the occurrence of these frustrating bugs and improve the overall quality and reliability of your software. Remember, prevention is always better than cure, and consistent attention to detail is crucial in eliminating the source of these insidious errors.
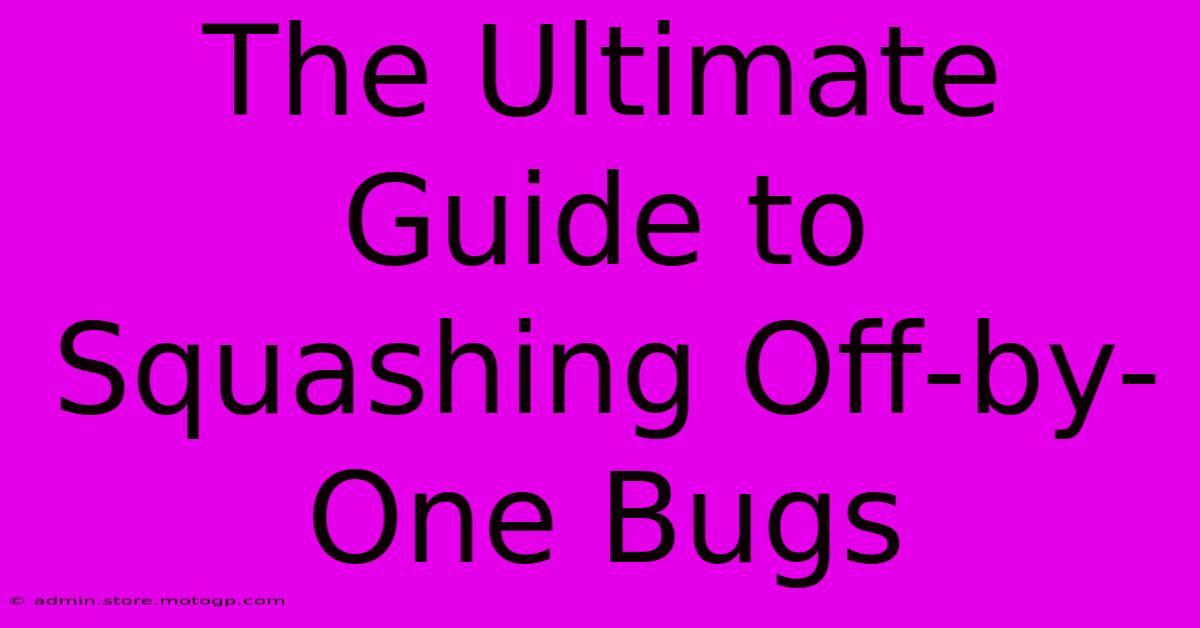
Thank you for visiting our website wich cover about The Ultimate Guide To Squashing Off-by-One Bugs. We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and dont miss to bookmark.
Featured Posts
-
Creating A Vision Safe Haven For Your Newborn
Feb 10, 2025
-
Experience The Magic Again Ouat Season 7 Is Here
Feb 10, 2025
-
Leyton House Limor The F1 Car That Could Have Won It All
Feb 10, 2025
-
Patricia Heatons Net Worth Lessons On Building Wealth
Feb 10, 2025
-
Unrivaled Speed And Comfort Explore The 2004 Citation X
Feb 10, 2025