The VBA Variable Revolution: Redefining Form Control Customization
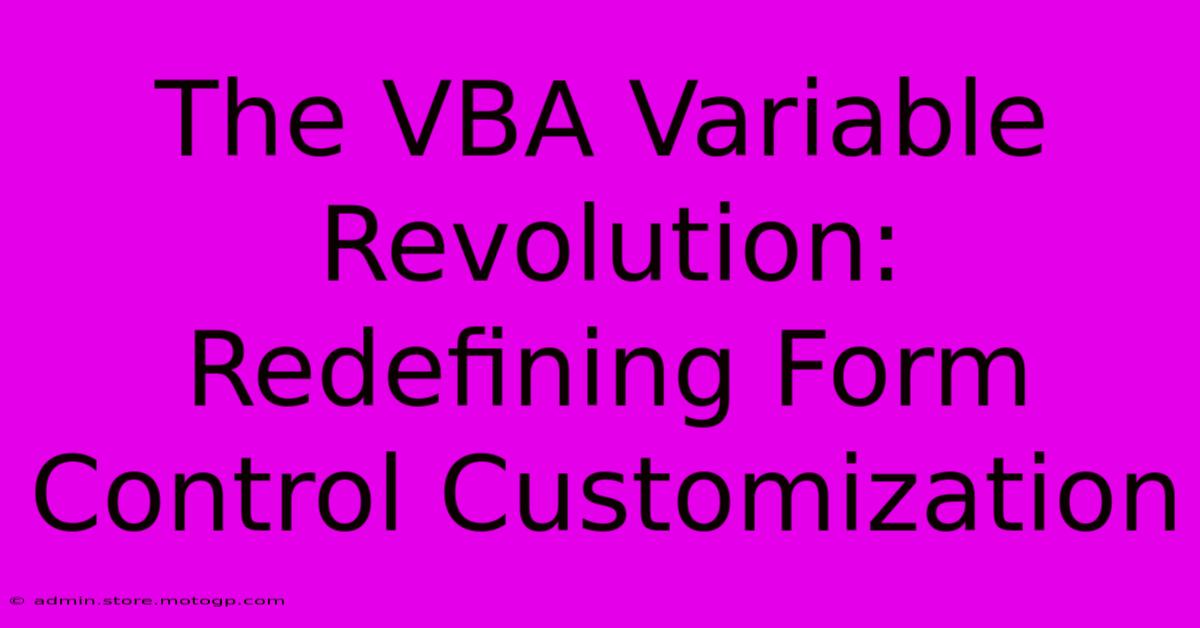
Table of Contents
The VBA Variable Revolution: Redefining Form Control Customization
Visual Basic for Applications (VBA) is a powerful tool that allows you to significantly enhance the functionality of Microsoft Office applications. One area where VBA truly shines is in customizing form controls. By leveraging the power of variables, you can take your form control manipulation to a whole new level, creating dynamic and responsive user interfaces. This article will explore how effectively using VBA variables revolutionizes form control customization.
Understanding the Power of Variables in VBA Form Control Customization
Before diving into specific examples, let's establish the fundamental role of variables. In VBA, variables act as containers that store data. This data can be anything from simple text strings to complex objects like form controls themselves. By assigning form controls to variables, you can easily manipulate their properties and events without repeatedly referencing their lengthy names. This makes your code cleaner, more efficient, and much easier to maintain.
Key Advantages of Using Variables:
-
Improved Code Readability: Instead of using long, cumbersome control names, you can use descriptive variable names that instantly clarify your code's purpose. For example, instead of
Me.CommandButton1.Visible = False
, you could usebtnSubmit.Visible = False
, making the intent instantly clear. -
Enhanced Code Maintainability: If you need to rename a control, you only need to change the variable assignment; you don't have to hunt through your code to update every instance of the control's original name.
-
Increased Code Reusability: Variables allow you to create reusable code modules. You can write functions or subroutines that operate on variables representing form controls, making your code more modular and efficient.
Practical Applications: Transforming Form Control Behavior
Let's explore some practical examples demonstrating how variables enhance form control customization:
1. Dynamically Enabling/Disabling Controls Based on User Input:
Imagine a form with multiple text boxes and a submit button. You want the submit button to remain disabled until all text boxes are filled. Using variables makes this remarkably straightforward:
Private Sub TextBox1_Change()
Dim txtBox1 As MSForms.TextBox
Dim txtBox2 As MSForms.TextBox
Dim btnSubmit As MSForms.CommandButton
Set txtBox1 = Me.TextBox1
Set txtBox2 = Me.TextBox2
Set btnSubmit = Me.CommandButton1
If Len(txtBox1.Value) > 0 And Len(txtBox2.Value) > 0 Then
btnSubmit.Enabled = True
Else
btnSubmit.Enabled = False
End If
End Sub
Private Sub TextBox2_Change()
'Same code as TextBox1_Change, demonstrating reusability
Dim txtBox1 As MSForms.TextBox
Dim txtBox2 As MSForms.TextBox
Dim btnSubmit As MSForms.CommandButton
Set txtBox1 = Me.TextBox1
Set txtBox2 = Me.TextBox2
Set btnSubmit = Me.CommandButton1
If Len(txtBox1.Value) > 0 And Len(txtBox2.Value) > 0 Then
btnSubmit.Enabled = True
Else
btnSubmit.Enabled = False
End If
End Sub
This code dynamically enables the submit button only when both text boxes contain data. Note the use of variables txtBox1
, txtBox2
, and btnSubmit
for improved readability and maintainability.
2. Creating Dynamically Populated Combo Boxes:
Suppose you need a combo box whose contents change based on a user's selection in another control. Using variables allows for elegant control of this dynamic population. A simplified example could involve populating a list of products based on a chosen category.
'Example code snippet illustrating dynamic population
Private Sub CategoryCombo_Change()
Dim category As String
Dim productCombo As MSForms.ComboBox
Set productCombo = Me.ProductCombo
category = Me.CategoryCombo.Value
' Code to populate productCombo based on the selected category (using category variable)
End Sub
3. Managing Complex Form Layouts with Ease:
In forms with numerous controls, managing their visibility, position, and other properties becomes much simpler with variables. You can group related controls into variables, making bulk operations (such as hiding or showing a section of the form) much more efficient.
Conclusion: Embrace the Variable Revolution
By incorporating variables into your VBA form control customization, you drastically improve code quality, maintainability, and overall efficiency. The examples shown above only scratch the surface of what's possible. As you become more proficient, you'll find that using variables is not just a good practice but an essential component of creating sophisticated and robust VBA-driven user interfaces. So, embrace the variable revolution and unlock the true potential of VBA for your form control needs.
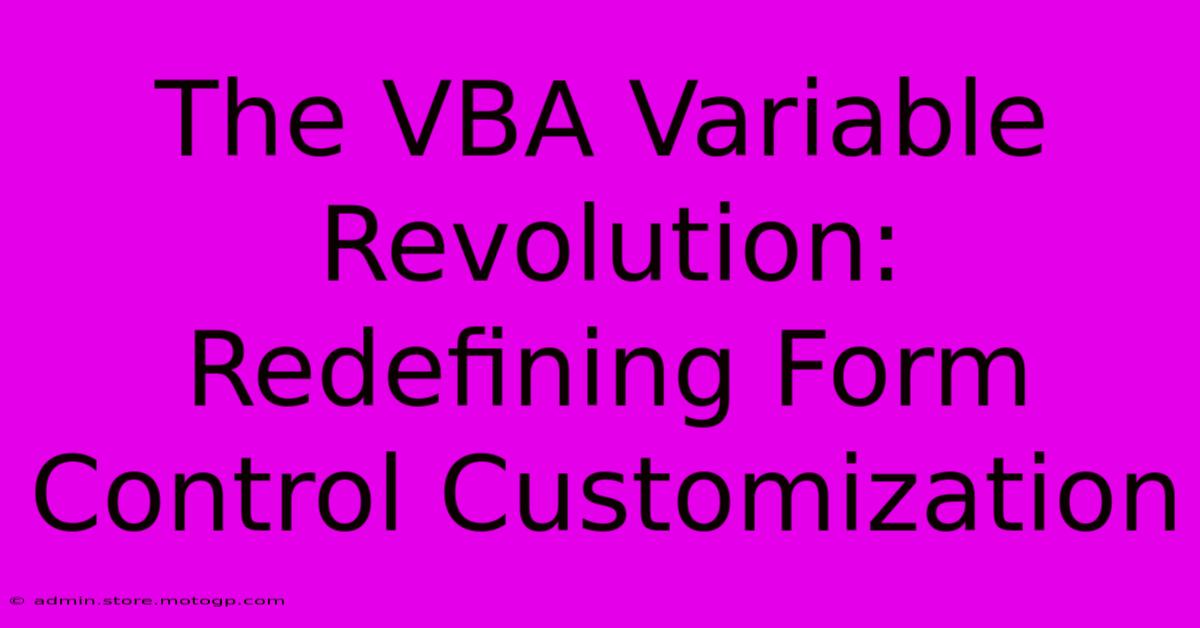
Thank you for visiting our website wich cover about The VBA Variable Revolution: Redefining Form Control Customization. We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and dont miss to bookmark.
Featured Posts
-
Huephoria The Ultimate Guide To Spray Rose Colors And Meanings
Feb 06, 2025
-
Unveiling The Truth What You Dont Know About No Soliciting Signs
Feb 06, 2025
-
Elevate Your Instagram Feed Discover The Art Of Flattering Posing
Feb 06, 2025
-
Ready For A Floral Extravaganza Heres The True Cost Of A Bohemian Green Bouquet
Feb 06, 2025
-
Digging Deep Delving Into The Surprising Cost Of A Boho Green Floral Masterpiece
Feb 06, 2025