Unlock The Secrets Of VBA Range Text Replacement: A Step-by-Step Odyssey
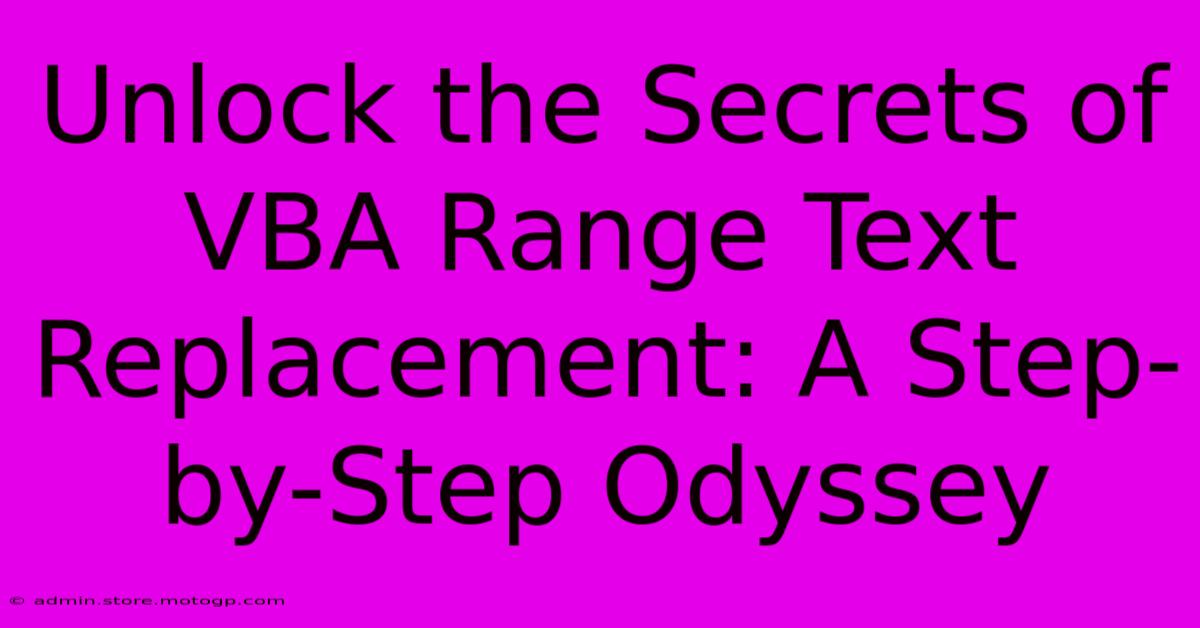
Table of Contents
Unlock the Secrets of VBA Range Text Replacement: A Step-by-Step Odyssey
Visual Basic for Applications (VBA) is a powerful tool within Microsoft Office applications, allowing automation of tasks that would otherwise be tedious and time-consuming. One such task is text replacement within a specified range of cells. This article will guide you through the process, uncovering the secrets of efficient VBA range text replacement, from simple substitutions to complex pattern matching. We'll cover various scenarios and provide you with adaptable code examples.
Understanding the Fundamentals: VBA's Replace
Function
At the heart of VBA text replacement lies the Replace
function. This function allows you to substitute occurrences of one string with another within a given text string. Its basic syntax is:
Replace(string, find, replace, [start], [count], [compare])
- string: The original text string.
- find: The substring to be replaced.
- replace: The substring to replace the
find
string. - start: (Optional) The starting position within the string to begin searching.
- count: (Optional) The number of occurrences to replace.
- compare: (Optional) Specifies the type of comparison (e.g., case-sensitive).
Replacing Text within a Single Cell
Let's start with the simplest scenario: replacing text within a single cell. The following code snippet demonstrates this:
Sub ReplaceTextInSingleCell()
Dim ws As Worksheet
Dim cell As Range
Set ws = ThisWorkbook.Sheets("Sheet1") 'Change "Sheet1" to your sheet name
Set cell = ws.Range("A1")
cell.Value = Replace(cell.Value, "old text", "new text", , , vbTextCompare) 'vbTextCompare ignores case
End Sub
This code selects cell A1 on Sheet1 and replaces all instances of "old text" with "new text," ignoring case sensitivity due to vbTextCompare
.
How to handle different comparison methods?
The compare
argument in the Replace
function allows for different comparison methods. vbBinaryCompare
performs a case-sensitive comparison, while vbTextCompare
is case-insensitive. Choosing the correct comparison method is crucial for accurate results. For instance, using vbBinaryCompare
would only replace "old text" and not "Old Text" or "OLD TEXT".
Replacing Text Across a Range of Cells
Replacing text across multiple cells requires looping through the range. The following code iterates through a range of cells (A1:A10) and performs the replacement:
Sub ReplaceTextInRange()
Dim ws As Worksheet
Dim cell As Range
Set ws = ThisWorkbook.Sheets("Sheet1")
For Each cell In ws.Range("A1:A10")
cell.Value = Replace(cell.Value, "old text", "new text", , , vbTextCompare)
Next cell
End Sub
This code efficiently handles multiple cells, applying the replacement to each one individually.
How to efficiently handle large datasets?
For extremely large datasets, processing cell by cell can be slow. Consider using arrays to improve performance. This involves reading the entire range into an array, processing the array, and then writing the array back to the sheet. This method significantly reduces interaction with the worksheet, leading to substantial speed improvements.
Handling More Complex Scenarios: Regular Expressions
For more complex text manipulation, VBA's regular expressions offer powerful pattern-matching capabilities. This allows you to replace text based on patterns rather than just exact string matches. You'll need to add a reference to the "Microsoft VBScript Regular Expressions 5.5" library.
Sub ReplaceTextWithRegex()
Dim ws As Worksheet
Dim cell As Range
Dim regex As Object
Dim matches As Object
Set ws = ThisWorkbook.Sheets("Sheet1")
Set regex = CreateObject("VBScript.RegExp")
regex.Pattern = "\b[Oo]ld\s[Tt]ext\b" ' Matches "old text" or "Old text" with word boundaries
regex.IgnoreCase = True
regex.Global = True
For Each cell In ws.Range("A1:A10")
If regex.Test(cell.Value) Then
cell.Value = regex.Replace(cell.Value, "new text")
End If
Next cell
End Sub
This code uses a regular expression to match "old text" (regardless of case) and replace it with "new text". The \b
ensures that only whole words are matched.
What are the limitations of using regular expressions for text replacement in VBA?
While powerful, regular expressions can be complex and require a good understanding of their syntax. Overly complex patterns can impact performance. Simple replacements are often best handled with the standard Replace
function for efficiency.
Conclusion
VBA provides versatile tools for text replacement within Excel. From simple single-cell replacements to sophisticated pattern matching using regular expressions, the methods described offer solutions for various scenarios. Understanding the strengths and limitations of each approach is vital for choosing the most efficient and accurate method for your specific needs. Remember to always back up your data before running any VBA code. Happy coding!
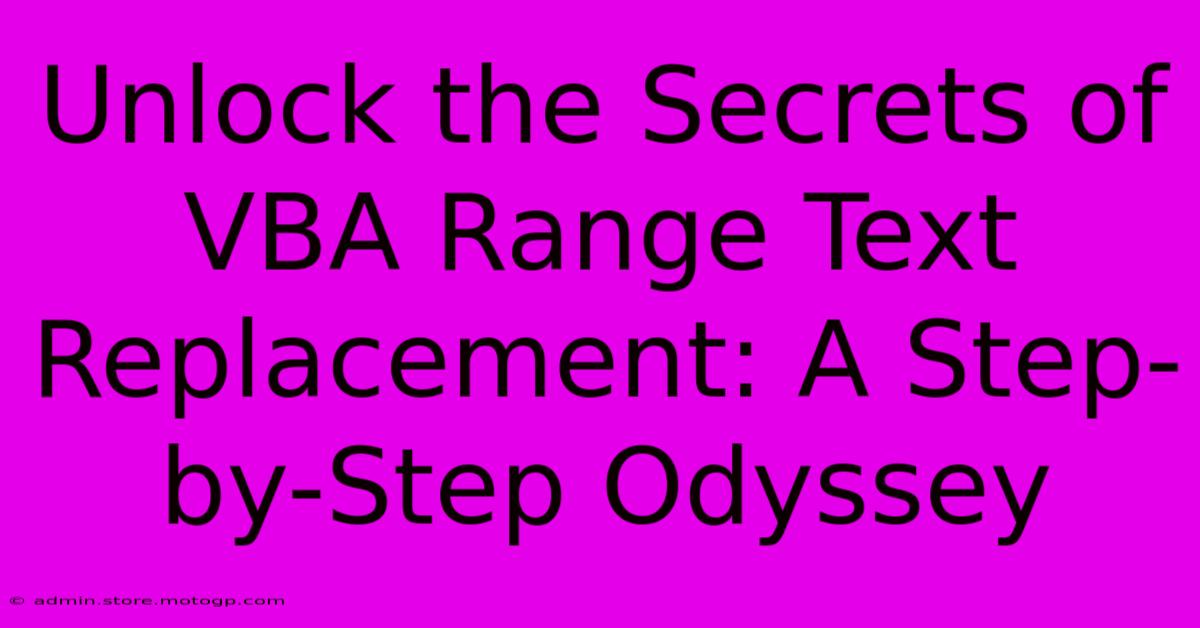
Thank you for visiting our website wich cover about Unlock The Secrets Of VBA Range Text Replacement: A Step-by-Step Odyssey. We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and dont miss to bookmark.
Featured Posts
-
The Lost Art Of Celtic Symbolism Unraveling The Hidden Meanings
Mar 05, 2025
-
Elevate Your Wedding With Fuchsia Bouquets The Perfect Hue For Unforgettable Moments
Mar 05, 2025
-
Rose Quartz Nirvana A Comprehensive Guide To Purchasing The Finest Gems Online
Mar 05, 2025
-
Reality Fractured Quantum Breaks Time Jumps Explored
Mar 05, 2025
-
The Calming Hue What Color Radiates Peace Like A Gentle Breeze
Mar 05, 2025