VBA's Label And Textbox Transformer: The Power Of Variables
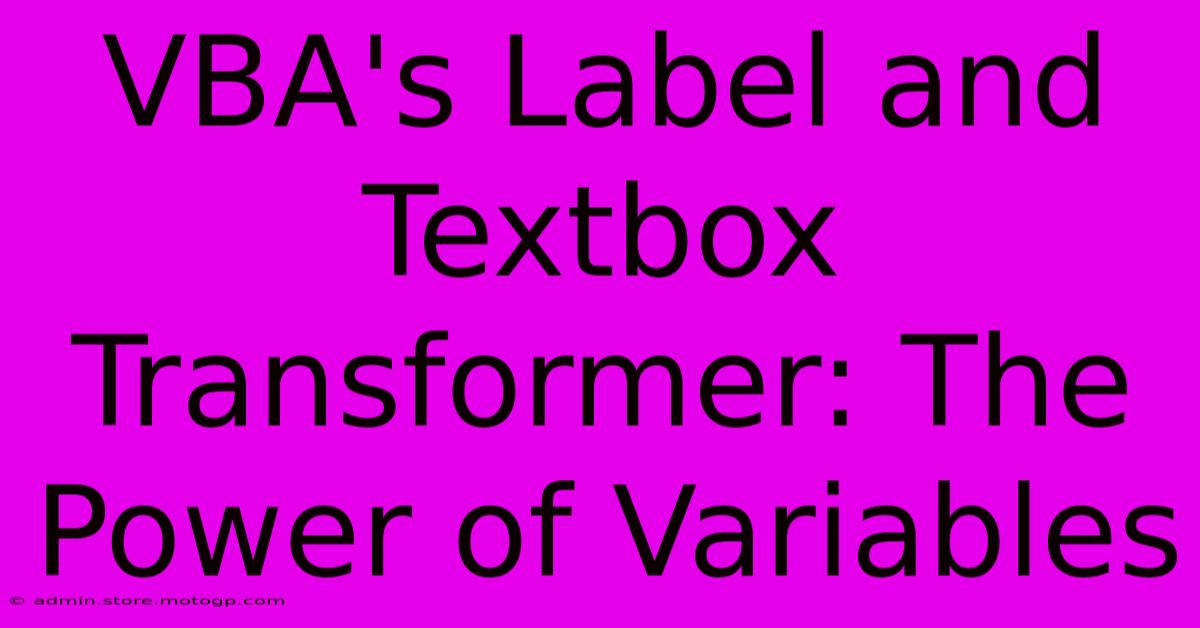
Table of Contents
VBA's Label and Textbox Transformer: The Power of Variables
Unlocking the true potential of your VBA forms requires mastering the dynamic interplay between labels, textboxes, and the power of variables. This article will guide you through leveraging variables to transform static forms into interactive and responsive interfaces. We'll explore how to seamlessly update label captions and textbox values, making your VBA applications more user-friendly and efficient.
Understanding the Foundation: Variables in VBA
Before diving into label and textbox manipulation, let's solidify our understanding of variables in VBA. Variables are containers that store data, allowing you to dynamically manage information within your code. They are essential for creating flexible and reusable code. Consider these key aspects:
- Declaration: Always declare your variables using the
Dim
statement. This improves code readability and helps prevent errors. For example:Dim myVariable As String
. - Data Types: Choose the appropriate data type (String, Integer, Boolean, etc.) based on the type of data your variable will hold. Selecting the correct data type is crucial for efficiency and error prevention.
- Scope: The scope of a variable determines where it's accessible within your code. Variables declared within a procedure (like a Sub or Function) are local; variables declared at the module level are accessible throughout the module.
Dynamically Updating Labels with Variables
Static labels limit the interactivity of your VBA forms. Using variables, you can change a label's caption based on user input or calculated values. This transforms a passive display into a dynamic feedback mechanism.
Here's how to update a label's caption using a variable:
Private Sub CommandButton1_Click()
Dim userName As String
userName = TextBox1.Value
'Update the label caption
Label1.Caption = "Welcome, " & userName & "!"
End Sub
In this example, the userName
variable stores the text entered in TextBox1
. The &
operator concatenates the welcome message with the username, updating Label1
's caption dynamically.
Beyond Simple Concatenation: Formatting and Calculations
You can go beyond simple string concatenation. Use variables to incorporate calculated results into your label captions:
Private Sub CommandButton1_Click()
Dim num1 As Integer, num2 As Integer, sum As Integer
num1 = TextBox1.Value
num2 = TextBox2.Value
sum = num1 + num2
Label1.Caption = "The sum is: " & sum
End Sub
This demonstrates how to perform calculations and display the result in a label. The possibilities are endless – you can incorporate any valid VBA expression to dynamically generate label captions.
Controlling Textboxes with Variables: Input Validation and More
Variables are equally powerful for managing textboxes. You can use them to pre-populate textboxes, validate user input, or even disable/enable textboxes based on application logic.
Pre-populating Textboxes
Private Sub UserForm_Initialize()
Dim defaultName As String
defaultName = "John Doe"
TextBox1.Value = defaultName
End Sub
This snippet pre-populates TextBox1
with "John Doe" when the userform initializes.
Input Validation: Ensuring Data Integrity
Variables assist in validating user input before it's processed further:
Private Sub CommandButton1_Click()
Dim age As Integer
If IsNumeric(TextBox1.Value) Then
age = CInt(TextBox1.Value)
If age > 0 And age < 120 Then
'Process valid age
Label1.Caption = "Valid age entered."
Else
MsgBox "Please enter a valid age (between 0 and 120)."
End If
Else
MsgBox "Please enter a numeric value for age."
End If
End Sub
This example checks if the input in TextBox1
is a number and within a valid range.
Advanced Techniques: Arrays and Collections
For more complex scenarios involving multiple labels and textboxes, consider using arrays or collections to manage them efficiently. This allows for looping and programmatic manipulation, reducing repetitive code.
Conclusion: Mastering the Dynamic Form
By integrating variables into your VBA code, you can transform your static forms into dynamic and responsive interfaces. This enhanced interactivity creates a more user-friendly experience and improves the overall efficiency of your VBA applications. Experiment with different techniques and explore the limitless possibilities offered by variables to create truly powerful and adaptable VBA forms. Remember to always thoroughly test your code and handle potential errors gracefully for a robust and reliable application.
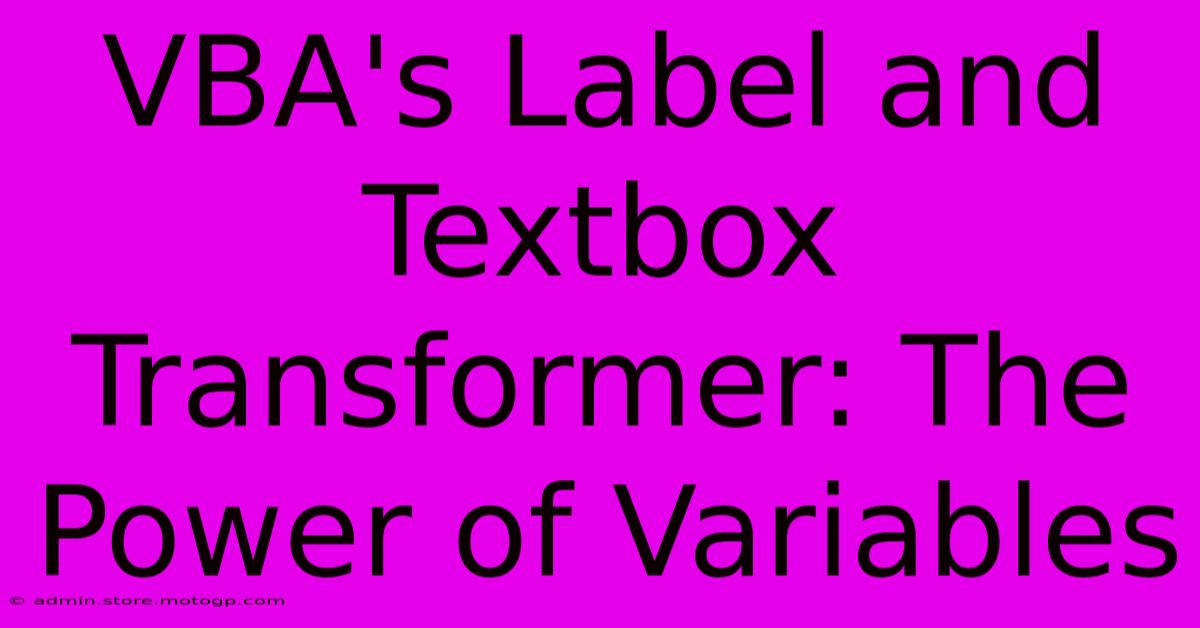
Thank you for visiting our website wich cover about VBA's Label And Textbox Transformer: The Power Of Variables. We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and dont miss to bookmark.
Featured Posts
-
Revolutionize Your Gaming Experience Console Extremes With Our Unbreakable Hdmi Cable
Feb 06, 2025
-
Tropical Twist Unlocking The Exotic Charms Of Yellow Spray Roses
Feb 06, 2025
-
You Wont Believe These Hilarious Football Player Names
Feb 06, 2025
-
Ascend To Excellence With Counters Upward Strokes
Feb 06, 2025
-
100 Polyester Shrinkage Mystery Revealed
Feb 06, 2025